Client-Side and Server-Side Issues: Causes and Solutions
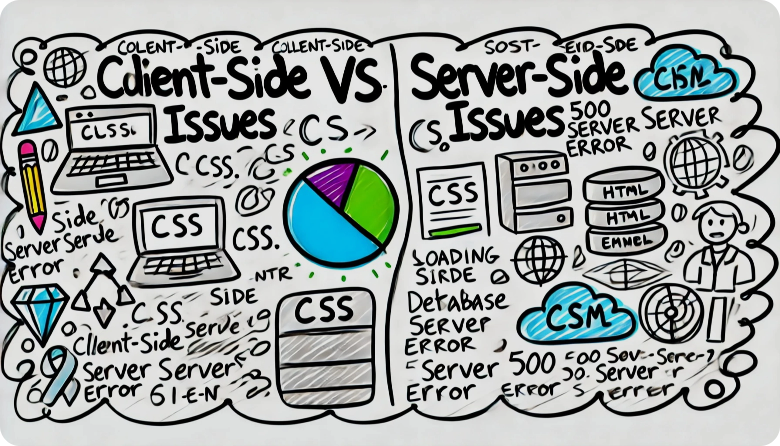
In web development, ensuring that your website runs smoothly across all devices and platforms is key to delivering a great user experience. But what happens when users start experiencing problems while interacting with your site? These problems typically fall into two categories: client-side and server-side issues.
Understanding these issues' differences is essential for developers and business owners because both can significantly impact a website's performance, usability, and security. In this blog post, we’ll examine client-side and server-side issues, how to identify and solve them, and what tools are available to help in the process.
What are Client-Side Issues?
Client-side issues are problems that occur in the user's browser or device when they try to interact with a website. These issues typically relate to how the website is displayed or functions in the browser. Client-side refers to everything that happens on the user’s end, from the HTML rendering to the execution of JavaScript.
Common Client-Side Issues:
1. Slow Page Load Time:
- Cause: Excessive use of large images, inefficient CSS, JavaScript files, or too many HTTP requests.
- Solution:
- Compress images and optimize their size without compromising quality.
- Minify CSS, JavaScript, and HTML files.
- Use lazy loading for images and videos.
- Leverage browser caching and content delivery networks (CDNs).
2. JavaScript Errors:
- Cause: Incompatible or poorly written JavaScript code can cause the website to fail in certain browsers or devices.
- Solution:
- Test the site in different browsers and devices.
- Use JavaScript linters (like ESLint) to catch syntax errors.
- Debug using browser developer tools.
3. Browser Compatibility Issues:
- Cause: Different browsers interpret code slightly differently, which can cause issues with how the site is displayed.
- Solution:
- Use feature detection and fallbacks in CSS.
- Regularly test the site in multiple browsers (Google Chrome, Mozilla Firefox, Safari, Edge).
- Utilize polyfills or modernizer libraries to handle outdated browser functionality.
4. Responsive Design Problems:
- Cause: Poorly implemented responsive design may cause the site to appear distorted or hard to navigate on mobile devices.
- Solution:
- Implement responsive design using CSS media queries and frameworks like Bootstrap.
- Test on various screen sizes using tools like Chrome Developer Tools or services like BrowserStack.
5. Cross-Origin Resource Sharing (CORS) Issues:
- Cause: A server may block access to resources if the request is made from a different domain, causing functionality like AJAX requests to fail.
- Solution:
- Adjust server settings to allow cross-origin requests.
- Use JSONP as a workaround for certain scenarios.
- Set proper headers like Access-Control-Allow-Origin on the server.
6. Unoptimized Web Fonts:
- Cause: Using multiple large font files can slow down page rendering and increase load times.
- Solution:
- Use system fonts or only load the necessary font weights and styles.
- Implement font-display: swap; to avoid blank text during font loading.
- Minimize the number of web fonts in use and prefer compressed formats like WOFF2.
7. Memory Leaks in JavaScript:
- Cause: Poorly written JavaScript code that holds onto memory even after it’s no longer needed.
- Solution:
- Regularly check and test your code using browser developer tools to detect memory leaks.
- Make sure to clean up unused variables and event listeners.
8. Blocking Resources (Render-Blocking JavaScript and CSS):
- Cause: JavaScript or CSS files that prevent the page from rendering until they’re fully loaded.
- Solution:
- Use asynchronous loading (async or defer attributes) for non-critical JavaScript files.
- Minify and combine CSS files to reduce HTTP requests.
- Implement critical CSS to load above-the-fold content faster.
9. Client-Side Caching Issues:
- Cause: Outdated resources are served from the cache, preventing the user from seeing the most recent updates.
- Solution:
- Use cache-busting techniques by appending versioning numbers to static assets.
- Set appropriate cache-control headers so that critical files are refreshed when necessary.
10. Web Accessibility Issues:
- Cause: A site that lacks accessibility features can be difficult for people with disabilities to navigate.
- Solution:
- Implement ARIA (Accessible Rich Internet Applications) attributes to improve assistive technology interactions.
- Ensure all images have alt attributes, and interactive elements can be navigated via keyboard.
- Use color contrast and font sizes that meet accessibility standards (WCAG).
11. Animations and Transitions Performance Issues:
- Cause: Excessive or poorly optimized CSS or JavaScript animations can lead to laggy or choppy performance on low-end devices.
- Solution:
- Use CSS animations instead of JavaScript whenever possible for better performance.
- Limit the number of elements being animated and avoid animating layout-affecting properties like width and height. Instead, animate properties like transform and opacity.
12. Client-Side Form Validation Errors:
- Cause: Poorly implemented form validation can lead to bad UX or security vulnerabilities.
- Solution:
- Implement proper validation for all input fields using HTML5 attributes like required, type, and pattern.
- Use JavaScript as a fallback to enhance form validation and prevent user mistakes.
Tools to Identify and Solve Client-Side Issues:
- Google Chrome Developer Tools: Essential for debugging and diagnosing problems like JavaScript errors and network-related performance issues.
- Lighthouse: A tool for auditing performance, accessibility, SEO, and more on your website.
- BrowserStack: Allows testing on real devices and browsers to ensure cross-browser compatibility.
- Pingdom Tools or GTMetrix: These tools help measure page speed and suggest performance optimizations.
- ESLint: A JavaScript linter that helps catch potential bugs and syntax errors in your code.
- PerfTool: A browser-based tool that provides real-time insights into site performance and can help diagnose client-side issues related to load time.
- CSSLint: Helps catch errors and inefficiencies in your CSS code, improving maintainability and performance.
- Fiddler: A network debugging tool that allows you to monitor HTTP requests and responses, helping identify client-side network issues.
- PageSpeed Insights: A Google tool that provides detailed performance diagnostics for your website and suggestions for optimization.
What are Server-Side Issues?
Server-side issues occur on the web server hosting the website. These issues are often related to how the server responds to requests from clients (users). If the server doesn’t respond properly or takes too long to process requests, it can lead to a poor user experience.
Common Server-Side Issues:
1. Slow Server Response Time:
- Cause: The server may be overloaded, have poor configuration, or face database issues.
- Solution:
- Optimize server configurations and code (e.g., by caching server responses).
- Scale server resources (RAM, CPU) or move to cloud hosting solutions with better load handling like AWS, Azure.
- Use load balancers to distribute traffic across multiple servers.
- Optimize database queries and indexes to reduce latency.
2. 500 Internal Server Error:
- Cause: Misconfigurations, server overload, or corrupt .htaccess files.
- Solution:
- Check server logs for error details.
- Fix any misconfigurations in server files (like .htaccess or Nginx/Apache configurations).
- Increase server resources or clear the cache if the error is due to overload.
3. 404 Not Found Error:
- Cause: The server cannot find the requested resource.
- Solution:
- Ensure that URLs are correct and resources exist on the server.
- Implement custom 404 error pages and redirect strategies for broken links.
4. Database Connection Issues:
- Cause: The server might fail to connect to the database due to incorrect credentials or the database being down.
- Solution:
- Check database server status.
- Verify connection credentials and permissions.
- Ensure the database isn’t overloaded by optimizing queries or upgrading hardware.
5. Security Vulnerabilities (SQL Injection, DDoS Attacks):
- Cause: Poorly written code or weak server security configurations.
- Solution:
- Use prepared statements to prevent SQL injection.
- Implement a firewall to filter traffic and detect threats.
- Utilize a web application firewall (WAF) and DDoS protection tools.
- Keep server software and plugins up to date to avoid exploits.
6. High Latency or Network Delays:
- Cause: Delays caused by long distances between users and the server or an overloaded network.
- Solution:
- Use a Content Delivery Network (CDN) to cache static content closer to users.
- Optimize your server's networking configurations and ensure the use of HTTP/2 to speed up content delivery.
7. Session Management Vulnerabilities:
- Cause: Poor handling of user sessions, which can lead to session hijacking or replay attacks.
- Solution:
- Implement secure, HTTP-only cookies with proper expiration times.
- Use secure tokens and strong hashing algorithms (e.g., JWTs) for session management.
- Regenerate session IDs after login to avoid session fixation attacks.
8. File Upload Vulnerabilities:
- Cause: Allowing users to upload files without proper validation can lead to security risks like malware or code execution on the server.
- Solution:
- Validate file types and sizes on both the client and server side.
- Store uploaded files outside the root web directory and scan them for malicious content.
- Implement rate limiting and size restrictions for uploaded files.
9. Data Loss or Corruption:
- Cause: Power outages, hardware failures, or software bugs can lead to data loss or corruption in databases.
- Solution:
- Implement regular backups with off-site storage.
- Ensure proper database replication and use RAID (Redundant Array of Independent Disks) configurations for storage redundancy.
- Use error-checking and data validation mechanisms during data writes.
10. Authentication and Authorization Flaws:
- Cause: Insecure login mechanisms or improper access control can lead to unauthorized access.
- Solution:
- Implement multi-factor authentication (MFA) for users and administrators.
- Ensure that roles and permissions are strictly enforced.
- Use OAuth2 or other secure authentication frameworks.
11. Memory Exhaustion:
- Cause: Server memory is overwhelmed by excessive requests or poor memory management.
- Solution:
- Use monitoring tools like New Relic or Datadog to track memory usage.
- Increase memory limits in your server configurations or optimize your application to reduce memory usage.
- Implement rate limiting and proper garbage collection in your code to avoid memory leaks.
12. API Rate Limiting Issues:
- Cause: Too many API requests can overwhelm the server, resulting in downtime or performance degradation.
- Solution:
- Implement API rate limiting to control the number of requests per user.
- Use caching mechanisms to reduce redundant API calls.
- Load balance API traffic across multiple servers.
13. DNS Configuration Issues:
- Cause: Incorrect DNS settings can cause the website to become unreachable or lead to significant downtime.
- Solution:
- Ensure your DNS records (A, CNAME, MX) are properly configured.
- Use services like Cloudflare to manage and optimize your DNS performance.
- Set up redundancy by using multiple DNS providers.
14. SSL/TLS Certificate Errors:
- Cause: Expired or misconfigured SSL certificates can result in browser warnings or blocked access.
- Solution:
- Regularly monitor and renew SSL/TLS certificates before expiration.
- Use automated tools like Let’s Encrypt to manage SSL certificates.
- Check your server’s SSL configuration using tools like SSL Labs to ensure proper implementation.
15. Overloaded Database Connections:
- Cause: An excessive number of concurrent database connections can slow down or crash the server.
- Solution:
- Implement connection pooling to limit the number of active database connections.
- Optimize your queries to reduce database load.
- Increase database connection limits or scale your database horizontally if needed.
Tools to Identify and Solve Server-Side Issues:
- New Relic or Datadog: Monitor server performance, response times, and bottlenecks in real-time.
- UptimeRobot: Monitors uptime and server availability and notifies you of any downtime.
- Apache or Nginx Logs: Server logs are invaluable for diagnosing issues related to server responses and configurations.
- Fail2Ban: Protects your server from brute force attacks and other types of intrusions.
- SQL Performance Monitor Tools (e.g., MySQL Workbench, pgAdmin): Help diagnose and optimize slow database queries.
- Nginx Amplify: A monitoring tool specifically designed for Nginx servers to track server performance and diagnose configuration issues.
- Loggly: A log management tool that allows you to aggregate server logs and monitor them for errors, warnings, and unusual patterns.
- Elastic Stack (ELK): A popular suite of tools (Elasticsearch, Logstash, and Kibana) for log management and monitoring of server infrastructure.
- Prometheus: An open-source tool that helps you monitor server metrics in real-time, track uptime, and identify performance bottlenecks.
Conclusion
Managing client-side and server-side issues is integral to maintaining an efficient, user-friendly website. As we’ve discussed, client-side issues such as slow page load times, browser compatibility problems, and JavaScript errors can negatively impact the user experience, leading to frustration and lost business opportunities. On the other hand, server-side issues like database errors, server downtime, and security vulnerabilities can cripple your website’s performance, making it inaccessible or insecure for users.
However, solving these issues isn’t just a technical task; it’s part of a broader approach to client satisfaction and business efficiency. By addressing client-side and server-side problems early, you ensure smooth website operations and build trust and reliability with your clients. Proactive maintenance, ongoing monitoring, and employing the right tools can drastically reduce the occurrence of these problems, saving both time and resources.
“Optimize your website, solve critical issues, and keep your online presence healthy! At Inboundsys, we tackle client-side and server-side issues before they affect your business. Get in touch today to see how our expert solutions can boost your website’s performance.

Thirumalesh Prasad C G (Thiru) is an entrepreneur, Founder, and CEO of Inboundsys. He has over 22 years of experience working for various multinational IT products and services companies in India and abroad. He was a significant member and worked as a user interface architect, designing the user interface for many web applications and products. In addition to running Inboundsys, he is an advisory board member in various other design studios and digital marketing agencies. He is a passionate blogger who loves writing on digital marketing, inbound marketing, lifestyle, philosophy, positive thinking, and motivation.